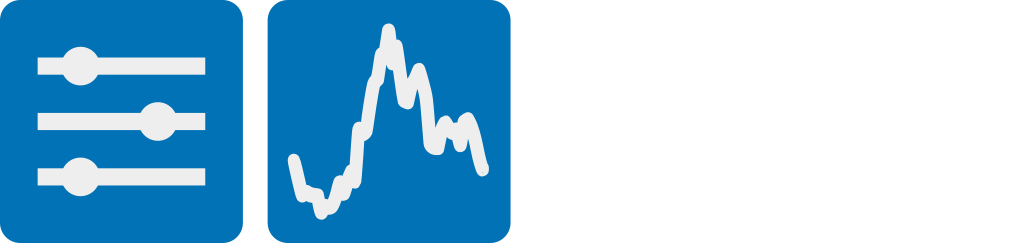
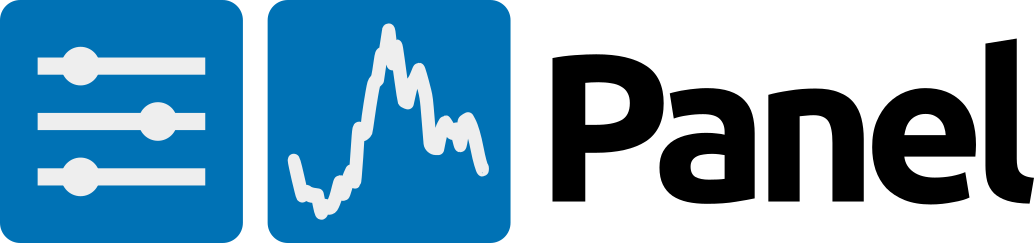
Overview#
The Powerful Data Exploration & Web App Framework for Python
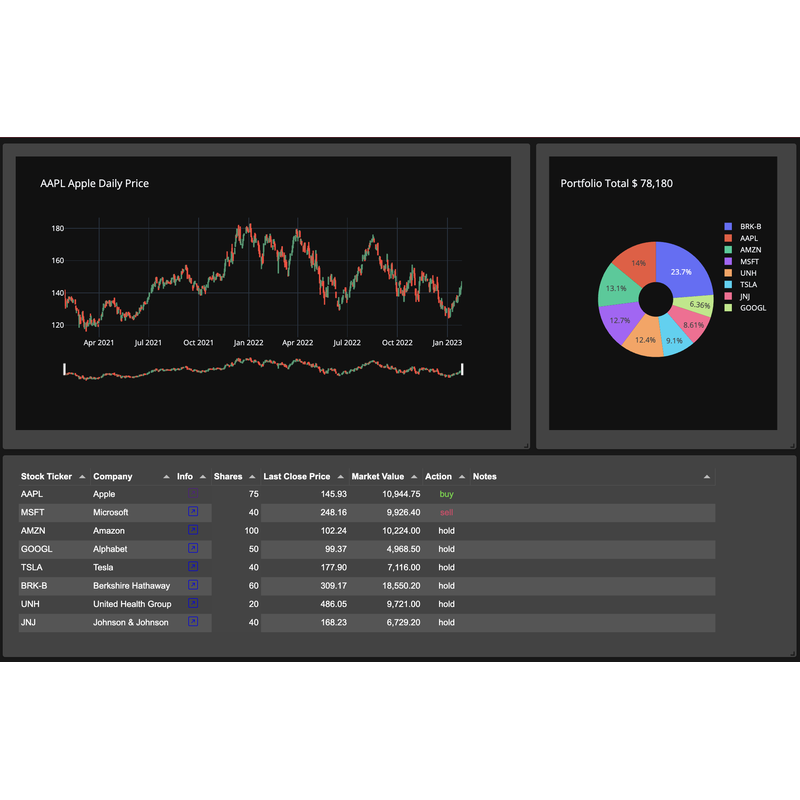
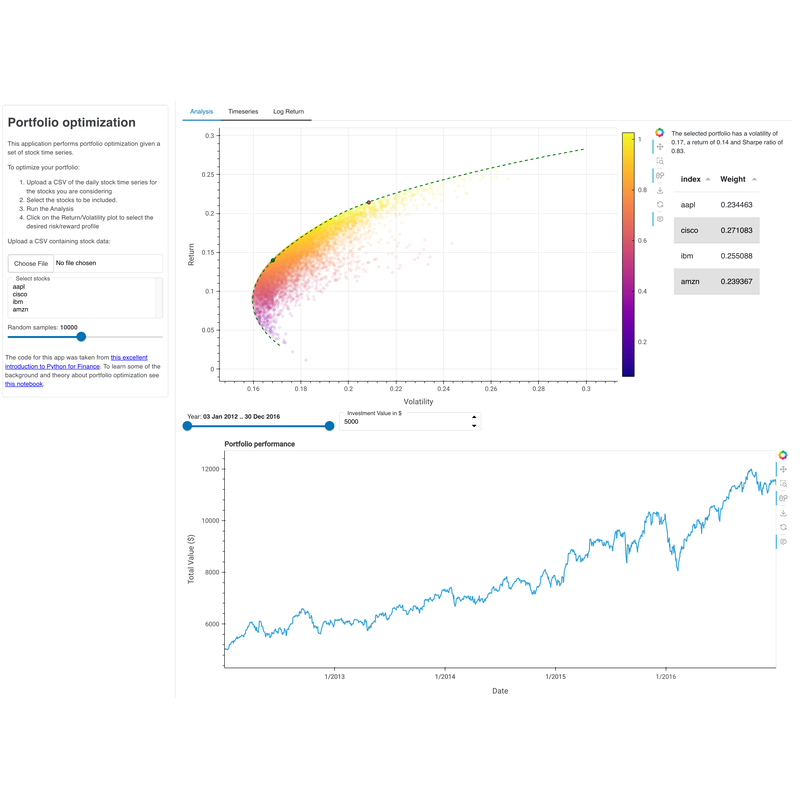
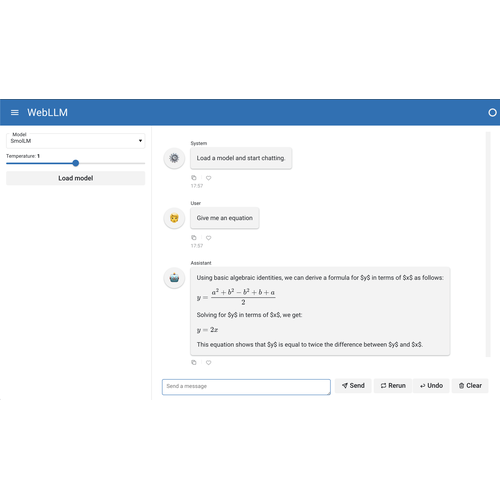
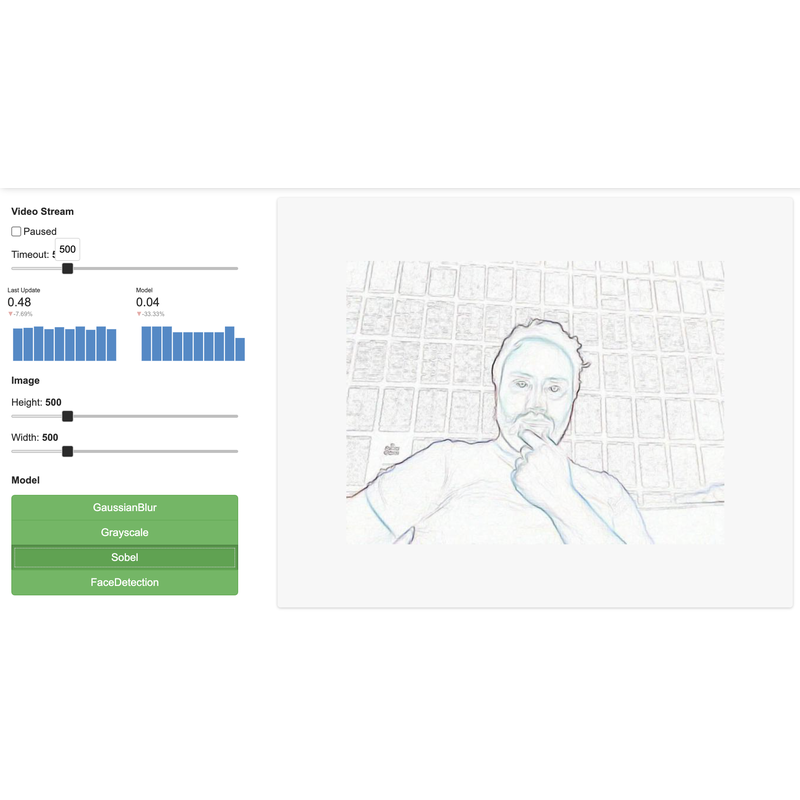
Panel is an open-source Python library designed to streamline the development of robust tools, dashboards, and complex applications entirely within Python. With a comprehensive philosophy, Panel integrates seamlessly with the PyData ecosystem, offering powerful, interactive data tables, visualizations, and much more, to unlock, visualize, share, and collaborate on your data for efficient workflows.
Its feature set includes high-level reactive APIs and lower-level callback-based APIs, enabling rapid development of exploratory applications and facilitating the creation of intricate, multi-page applications with extensive interactivity.
Panel is a proud member of the HoloViz ecosystem, providing a gateway to a cohesive suite of data exploration tools.
Panel makes it simple to:
Develop in your favorite editor or notebook environment
Combine the PyData tools and plotting libraries that you know and love
Iterate quickly to develop data tools, dashboards, and complex apps
Collaborate across skill levels and tool preferences
Add advanced bi-directional communication to your data apps
Create interactive big data applications with crossfiltering
Create high-performing, streaming data applications
Create data apps that can run entirely in the browser
Create polished, performant, secure, and production-ready web applications
Enjoying Panel? Show your support with a GitHub star — it’s a simple click that means the world to us and helps others discover it too! ⭐️
Learn Panel#
The getting started guide will get you set up with Panel and provide a basic overview of the features and strengths of Panel.
Through guided steps and activities, the tutorials will help you acquire the skills and knowledge to use Panel.
Introduces you to some of the core concepts behind Panel and some of the advanced features that make Panel such a powerful library.
Use Panel#
The Component Gallery showcases Panel’s components and their essential reference guides, offering users vital usage information.
How-to guides provide step-by-step recipes for solving essential problems and tasks that arise during your work.
The Panel API Reference Manual provides an extensive guide covering Panel’s methods and parameters.
For usage questions or technical assistance, please head over to Discourse or our Discord server. If you have any issues, feature requests, or wish to contribute, you can visit our GitHub site.
Sponsors#
The Panel project is grateful for the sponsorship by the organizations and companies below:
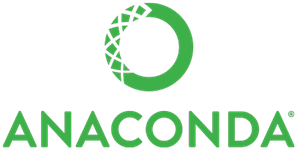
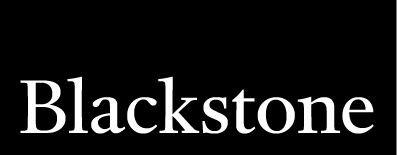
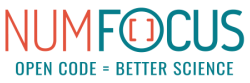