Customize Template Theme#
This guide addresses how to customize the theme of a template.
Prerequisites
The How to > Set a Template guide demonstrates how to set a template for a deployable app.
To implement a custom template theme you should declare a generic class and a specific class implementation for all the templates that should be supported, e.g. here is an example of what the definition of a DarkTheme
might look like for the MaterialTemplate
.
import param
import panel as pn
from panel.template.theme import Theme
from bokeh.themes import DARK_MINIMAL
class DarkTheme(Theme): # generic class
"""
The DarkTheme provides a dark color palette
"""
bokeh_theme = param.ClassSelector(class_=(Theme, str), default=DARK_MINIMAL)
class MaterialDarkTheme(DarkTheme): # specific class
# css = param.Filename() Here we could declare some custom CSS to apply
# This tells Panel to use this implementation
_template = pn.template.MaterialTemplate
Once these classes are created, the themes can be imported and applied to a template. We will use the generic DarkTheme
that is shipped with Panel to simplify the example below:
app.py
import hvplot.pandas
import numpy as np
import pandas as pd
import panel as pn
from panel.template import DarkTheme
# Data and Widgets
xs = np.linspace(0, np.pi)
freq = pn.widgets.FloatSlider(name="Frequency", start=0, end=10, value=2)
phase = pn.widgets.FloatSlider(name="Phase", start=0, end=np.pi)
# Interactive data pipeline
def sine(freq, phase):
return pd.DataFrame(dict(y=np.sin(xs*freq+phase)), index=xs)
dfi_sine = hvplot.bind(sine, freq, phase).interactive()
template = pn.template.MaterialTemplate(title='Material Dark', theme=DarkTheme)
template.sidebar.append(freq)
template.sidebar.append(phase)
template.main.append(
pn.Card(dfi_sine.hvplot(min_height=400).output(), title='Sine')
)
template.servable();
Now, we can activate this app on the command line:
panel serve app.py --show --dev
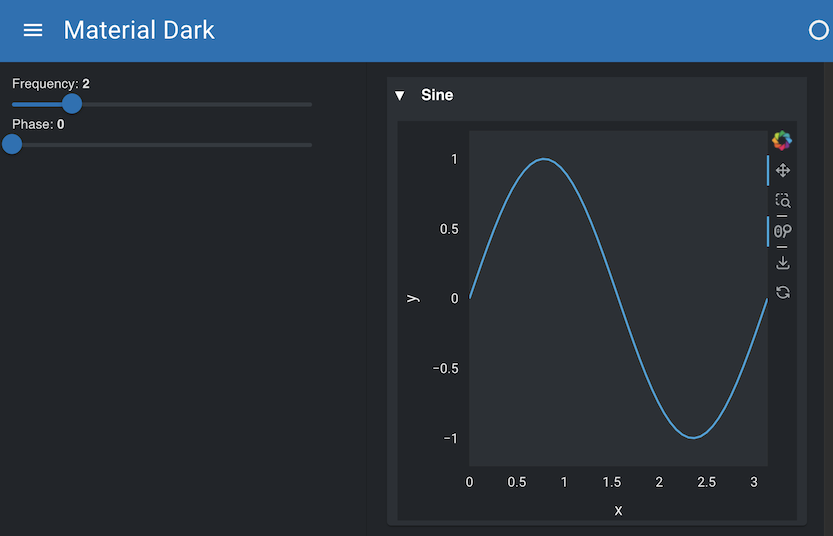